Optimizing Your Image Background Removal API
When you send extremely high-resolution images to our API, the long transfer times and redundant server-side resizing can slow down processing. Here’s a solution to streamline the process while maintaining high-quality output.
The Background
Our deep learning model resizes the image for alpha matte prediction, meaning the original high resolution isn’t used for processing, but is needed for the final output.
The Proposed Solution
Instead of uploading a full-resolution image, you can resize your images on the client side to the model’s optimal dimensions (where the longest side is 1024px). The API returns an alpha matte, which users can then merge with the original high-resolution image, preserving quality.
Implementation Overview
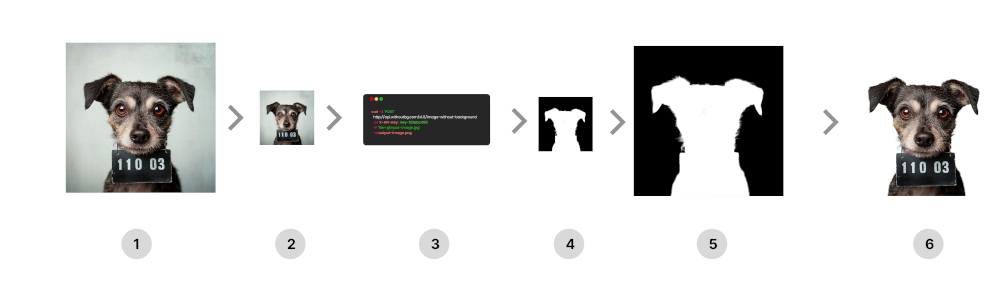
- (1) Original Image: The full-resolution image.
- (2) Client-Side Resizing (Client Side): Downscale the original image to the optimal dimensions using browser-based libraries (e.g., Pica or the Canvas API) before uploading.
- (3) API Request Formation: The resized image is sent to the API, ensuring faster uploads and efficient processing.
- (4) Alpha Matte Prediction (Server Side)
- (5) Alpha Matte Resized (Client Side): The predicted alpha matte is resized to the original image dimensions.
- (6) Client-Side Merging (Client Side): Resize the alpha matte to match the original image resolution image (e.g. using Fabric.js or the Canvas API), and apply the matte as an alpha channel. The output is a high-resolution image with the background effectively removed.
Example Code
This is how you can implement the solution in Python and PHP:
import requests
import json
import base64
from io import BytesIO
from PIL import Image
import numpy as np
def resize_image_for_api(image_path, max_size=1024):
"""Resize image maintaining aspect ratio with longest side = max_size"""
img = Image.open(image_path)
original_size = img.size
# Calculate new size maintaining aspect ratio
ratio = max_size / max(img.size)
new_size = tuple(int(dim * ratio) for dim in img.size)
# Resize image
resized_img = img.resize(new_size, Image.LANCZOS)
# Convert to bytes
img_byte_arr = BytesIO()
resized_img.save(img_byte_arr, format='PNG')
img_byte_arr = img_byte_arr.getvalue()
return img_byte_arr, original_size
def get_alpha_matte(image_path, api_key):
# Resize image for API and get original dimensions
resized_image_bytes, original_size = resize_image_for_api(image_path)
encoded_string = base64.b64encode(resized_image_bytes).decode('utf-8')
# Prepare the API request
url = "https://api.withoutbg.com/v1.0/alpha-channel-base64"
headers = {
"Content-Type": "application/json",
"X-API-Key": api_key
}
payload = json.dumps({"image_base64": encoded_string})
# Make the API call
response = requests.post(url, headers=headers, data=payload)
response.raise_for_status()
# Get alpha matte and resize to original dimensions
alpha_base64 = response.json()["alpha_base64"]
alpha_bytes = base64.b64decode(alpha_base64)
alpha_img = Image.open(BytesIO(alpha_bytes))
# Resize alpha matte to original image dimensions
alpha_img = alpha_img.resize(original_size, Image.LANCZOS)
return alpha_img
def remove_background(image_path, api_key, output_path):
# Open original image and convert to RGBA if needed
original_img = Image.open(image_path)
if original_img.mode != 'RGBA':
original_img = original_img.convert('RGBA')
# Get alpha matte
alpha_img = get_alpha_matte(image_path, api_key)
# Apply alpha channel to original image
original_img.putalpha(alpha_img)
# Create new image with transparent background
transparent_img = Image.new('RGBA', original_img.size, (0, 0, 0, 0))
transparent_img.paste(original_img, (0, 0), original_img)
# Save result
transparent_img.save(output_path, 'PNG')
# Usage
api_key = "YOUR_API_KEY"
image_path = "path/to/your/large_image.jpg"
output_path = "path/to/output/transparent.png"
remove_background(image_path, api_key, output_path)
Benefits
- Faster API Response: Smaller image files mean quicker uploads and reduced latency.
- Reduced Server Load: Processing smaller images optimizes resource usage.
- High-Quality Output: Merging the alpha matte with the original image preserves all high-resolution details.
Conclusion
By resizing images on the client side and merging the returned alpha matte with the original high-resolution image, you can achieve both speed and quality. This method benefits users with faster API responses and optimal resource usage, ensuring a seamless background removal process.